
📅 2017-02-06
本文内容已过期
我们已经部署了Ceph RGW服务,并使用管理工具radosgw-admin
创建了S3用户,本篇我们尝试使用S3 API访问Ceph RGW。
在开始之前先了解一下Amazon S3。
Amazon S3
#
Amazon S3(即Amazon Simple Storage Service) 是一种面向 Internet 的存储服务,Amazon还提供了S3 REST API可随时在 Web 上的任何位置存储和检索的任意大小的数据,同时提供Java、Python、Golang等各种语言的的SDK。
而Ceph RGW兼容绝大部分S3 Api,我们先熟悉一下S3服务的一些基本概念。
...📅 2017-02-05
Ceph RGW简介
#
Ceph RGW(即RADOS Gateway)是Ceph对象存储网关服务,是基于LIBRADOS接口封装实现的FastCGI服务,对外提供存储和管理对象数据的Restful API。
对象存储适用于图片、视频等各类文件的上传下载,可以设置相应的访问权限。目前Ceph RGW兼容常见的对象存储API,例如兼容绝大部分Amazon S3 API,兼容OpenStack Swift API。
...📅 2017-02-05
RBD简介
#
Ceph可以同时提供对象存储RADOSGW、块存储RBD、文件系统存储Ceph FS。
RBD即RADOS Block Device的简称,RBD块存储是最稳定且最常用的存储类型。RBD块设备类似磁盘可以被挂载。
RBD块设备具有快照、多副本、克隆和一致性等特性,数据以条带化的方式存储在Ceph集群的多个OSD中。
...📅 2017-02-05
系统运行级别
#
Linux系统的运行级别决定系统在哪种模式下运行,通过0~6这7个数字来表示运行级别。
系统运行级别:
- 0 - 关机: 运行级别设置成0,开机后就会进入关机状态。当运行级别切换到0时,会立即停止正在运行的服务,并关闭系统电源。
- 1 - 单用户模式: 无网络链接,不运行守护进程。主要用于系统的维护,只允许root用户登录。
- 2 - 本地多用户模式: 无网络链接,不运行守护进程。
- 3 - 完全多用户文本模式: 正常启动系统及相关服务。
- 4 - 用户自定义: 保留,未使用,主要是为开发人员定制功能,例如用于单片机或其他系统的开发和应用。
- 5 - 多用户图形系统: 该模式与3基本相同,除了文本模式之外还有图形界面
- 6 - 重启: 该级别是系统重启模式,系统在进入该级别后会立即重新启动
CentOS7系统运行级别
#
CentOS 7系统运行级别使用systemd的target替换了sysvinit,每个运行级别都有一个自己的target文件,可以在/lib/systemd/system目录下看到(实际上这些运行级别的target文件是一个软连接文件)
...📅 2017-02-04
查看IP转发功能的状态,若net.ipv4.ip_forward为0,表示禁止进行ip转发。
1sysctl net.ipv4.ip_forward
2net.ipv4.ip_forward = 0
修改 /etc/sysctl.conf:
执行如下命令使修改生效:
1sysctl -p /etc/sysctl.conf
📅 2017-02-03
RADOS简介
#
从Ceph官方文档摘录的架构图如下。RADOS是Ceph中最关键的部分,RADOS是一个支持海量对象的分布式对象存储。
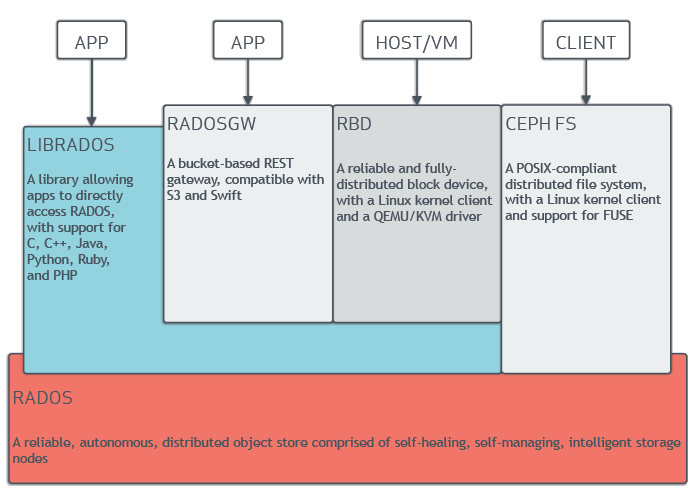
RADOS主要由两部分组成:
- Monitor节点集群:由少量的Monitor组成的小规模集群,负责管理的Map。Cluster Map是整个RADOS系统的关键数据结构,包含集群中全部成员、关系、属性等信息以及管理数据的分发。
- OSD节点集群:由大规模的OSD(Object Storage Device)组成的集群,负责存储所有的对象数据。
在物理结构上RADOS是由大量的存储节点组成,每个节点拥有自己的CPU、内存、硬盘、网络等硬件资源,并运行着操作系统和文件系统,OSD集群就是这些存储节点。、
而节点管理和数据分发策略都由Monitor负责,并为Client提供存储接口。
...📅 2017-02-03
系统环境
#
安装elasticsearch
#
📅 2017-02-02
Kubernetes中的Secret资源可以用来存储密码、Token、秘钥等敏感数据。
将这些敏感信息保存在Secret中,相对于暴露到Pod、镜像中更加的安全和灵活。
1.Kubernetes Secret的类型
#
Kubernetes内置了三种类型的Secret:
1.1 Service Account Secret
#
为了能从Pod内部访问Kubernetes API,Kubernetes提供了Service Account资源。
Service Account会自动创建和挂载访问Kubernetes API的Secret,会挂载到Pod的 /var/run/secrets/kubernetes.io/serviceaccount目录中。
关于这种类型的Secret我们这里先不展开。
...📅 2017-02-02
环境准备
#
- 机器(操作系统 CentOS)
- 192.168.61.30 c0 - admin-node, deploy-node
- 192.168.61.31 c1 - mon
- 192.168.61.32 c2 - osd.1
- 192.168.61.33 c3 - osd.2
配置各个节点的host文件:
1192.168.61.31 c0
2192.168.61.31 c1
3192.168.61.32 c2
4192.168.61.33 c3
管理节点c0上配置Ceph yum源 /etc/yum.repos.d/ceph.repo
1[ceph-noarch]
2name=Ceph noarch packages
3baseurl=https://download.ceph.com/rpm-jewel/el7/noarch
4enabled=1
5priority=2
6gpgcheck=1
7gpgkey=https://download.ceph.com/keys/release.asc
更新软件库并安装 ceph-deploy
...📅 2017-02-01
Ceph简介
#
Ceph是一个分布式存储系统,可以提供块存储RBD(Rados Block Device),对象存储RADOSGW(Rados Grateway)、文件系统存储(Ceph Filesystem)。
Ceph消除了对系统单一中心节点的依赖,实现了无中心结构的设计思想。
...